Studio Visual Editor
In the Global Elements Tab of your MUnit Test Suite, go to your Import (Configuration) element, and double-click it to show the Global Element Properties:
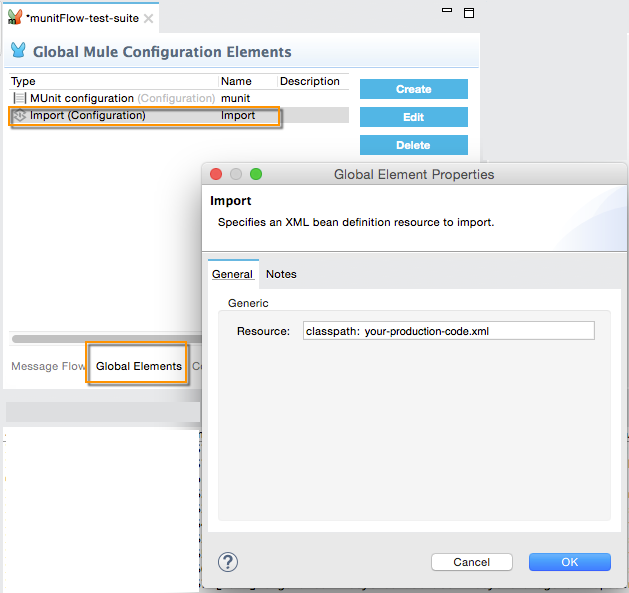
Edit the Resource: field with the reference of the .xml file name of the application you are testing.
XML or Standalone Editor
<spring:beans>
<spring:import resource="classpath:your-production-code.xml"/>
</spring:beans>