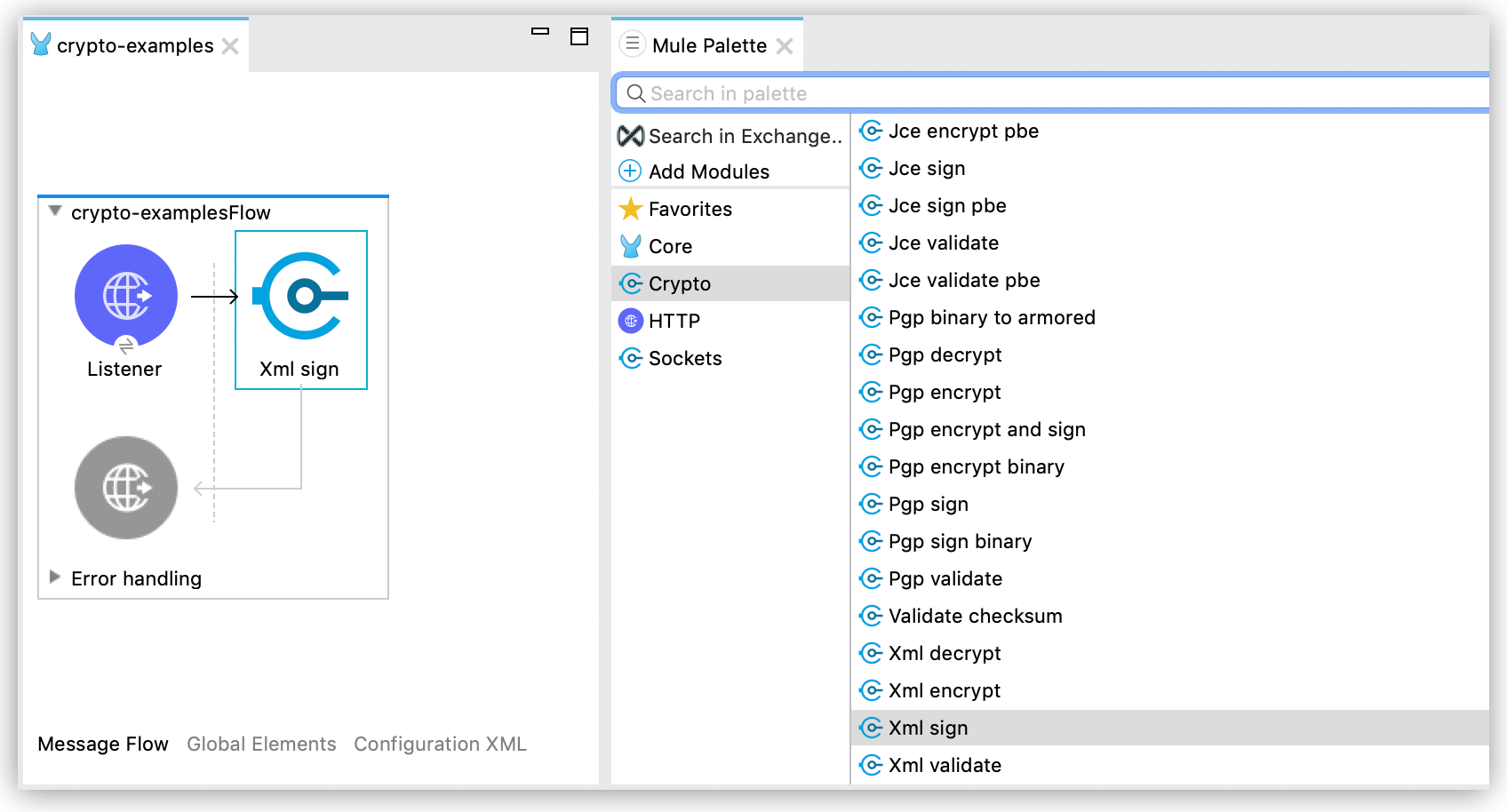
XML Cryptography
Configure XML Encryption from Anypoint Studio
To configure XML encryption from Anypoint Studio, follow these steps:
-
From the Mule palette, add Crypto to your project.
See Install the Extension for instructions.
-
Select the desired operation, and drag the component to the flow:
-
Open the component properties and select an existing Module configuration, or create a new one by specifying the Keystore, Type (JKS, JCEKS, PKCS12) and Password.
You can also add symmetric or asymmetric key information to be used in the sign operations:
-
Configure Key selection by using a Key id value previously defined in the module configuration, or define a new one for this operation:
-
Select Digest Algorithm, Canonicalization Algorithm, Type, and Element path.
Encrypting
This example configures a keystore that contains a symmetric key that will later be used for encryption.
<crypto:jce-config name="symmetricConfig" keystore="secret.jks" password="mule1234" type="JCEKS">
<crypto:jce-key-infos>
<crypto:jce-symmetric-key-info keyId="mySymKey" alias="aes128test" password="mule1234"/>
</crypto:jce-key-infos>
</crypto:jce-config>
In the next example, the XML encrypt operation is used to encrypt a specific element of the XML document.
<crypto:xml-encrypt config-ref="symmetricConfig" keyId="mySymKey" algorithm="AES_CBC" elementPath="//song"/>
The elementPath
is an XPath expression that identifies the element to encrypt.
Depending on your needs, you can use a symmetric or asymmetric key for encrypting an XML document.
Decrypting
<crypto:jce-config name="jceConfig" keystore="keystore.jks" password="mule1234">
<crypto:jce-key-infos>
<crypto:jce-symmetric-key-info keyId="mySymKey" alias="aes128test" password="mule1234"/>
<crypto:jce-asymmetric-key-info keyId="myAsymKey" alias="test1" password="test1234"/>
</crypto:jce-key-infos>
</crypto:jce-config>
In the next example, the XML decrypt operation (crypto:xml-decrypt
) is used to decrypt an XML document. The operation uses the asymmetric key stored in the referenced keystore.
<crypto:xml-decrypt config-ref="jceConfig" keyId="myAsymKey"/>
Depending on your needs, you can use a symmetric or asymmetric key for decryption.
Signing
<crypto:jce-config name="asymmetricConfig" keystore="keystore.jks" password="mule1234">
<crypto:jce-key-infos>
<crypto:jce-asymmetric-key-info keyId="myAsymKey" alias="test1" password="test1234"/>
</crypto:jce-key-infos>
</crypto:jce-config>
The next example uses the asymmetric key to sign an XML document by creating an XML envelope and inserting the signature inside the content that is being signed.
<crypto:xml-sign config-ref="asymmetricConfig" keyId="myAsymKey" type="ENVELOPING" digestAlgorithm="SHA256" elementPath="/PurchaseOrder/Buyer"/>
In the next example, a detached XML signature is created based on an element of the XML document. Instead of being inserted in the signed content, the detached signature is returned as a separate XML element.
<crypto:xml-sign config-ref="asymmetricConfig" keyId="myAsymKey" type="DETACHED" digestAlgorithm="SHA256" elementPath="/PurchaseOrder/Buyer"/>
Validating a Signature
<crypto:jce-config name="asymmetricConfig" keystore="keystore.jks" password="mule1234">
<crypto:jce-key-infos>
<crypto:jce-asymmetric-key-info keyId="myAsymKey" alias="test1" password="test1234"/>
</crypto:jce-key-infos>
</crypto:jce-config>
In the next example, the asymmetric key is used to validate the signature of the XML element specified by the elementPath
XPath expression.
<crypto:xml-validate config-ref="asymmetricConfig" keyId="myAsymKey" elementPath="/PurchaseOrder/Buyer"/>
If the document has multiple signatures, set elementPath
to select the signature to validate. Specify a signed element using an XPath expression to validate the signature for that element.
Targeting a Custom Namespace by Using elementPath
To sign or validate an XML element that is inside a custom namespace, specify the namespace by using the XPath functions: namespace-uri and local-name.
For example, consider the following document:
<soap:Envelope xmlns:soap="http://www.w3.org/2003/05/soap-envelope/">
<FirstElement>
<!-- Some content -->
</FirstElement>
<SecondElement>
<!-- Additional content -->
</SecondElement>
</soap:Envelope>
To target the content of FirstElement
inside soap:Envelope
, you specify the xmlns:soap
namespace. The XML schema for xmlns:soap
is defined in http://www.w3.org/2003/05/soap-envelope/
.
The following example shows an xml-sign
operation configured to sign FirstElement
:
<crypto:xml-sign
config-ref="asymmetricConfig"
keyId="myAsymKey"
type="DETACHED"
digestAlgorithm="SHA256"
elementPath="/*[namespace-uri()='http://www.w3.org/2003/05/soap-envelope/' and local-name()='Envelope']/FirstElement"/>
Note that the elementPath
expression specifies the xmlns:soap
namespace.
Reference
Module Configuration
JCE Configuration for Java keystores and inline keys to sign or encrypt XML documents or elements.
Parameters
Name | Type | Description | Default Value | Required |
---|---|---|---|---|
Name |
String |
The name for this configuration. Connectors reference the configuration with this name. |
x |
|
Keystore |
String |
Path to the keystore file. |
|
|
Type |
Enumeration, one of:
|
Type of the keystore. |
|
|
Password |
String |
Password for unlocking the keystore. |
|
|
Jce Key Infos |
Array of One of: |
List of keys to be considered, with internal IDs for referencing them. |
|
|
Expiration Policy |
Configures the minimum amount of time that a dynamic configuration instance can remain idle before the runtime considers it eligible for expiration. This does not mean that the platform will expire the instance at the exact moment that it becomes eligible. The runtime will actually purge the instances when it sees it fit. |
|
Xml Decrypt Operation
<crypto:xml-decrypt>
Decrypts the XML document.
Parameters
Name | Type | Description | Default Value | Required |
---|---|---|---|---|
Configuration |
String |
The name of the configuration to use. |
x |
|
Content |
Binary |
the document to decrypt |
|
|
Streaming Strategy |
|
Configure if repeatable streams should be used and their behavior |
|
|
Key Id |
String |
The key ID, as defined in the JCE configuration. |
|
|
Jce Key Info |
An inline key definition. |
|
||
Target Variable |
String |
The name of a variable on which the operation's output will be placed |
|
|
Target Value |
String |
An expression that will be evaluated against the operation's output and the outcome of that expression will be stored in the target variable |
|
|
Xml Encrypt Operation
<crypto:xml-encrypt>
Encrypt the XML document.
Parameters
Name | Type | Description | Default Value | Required |
---|---|---|---|---|
Configuration |
String |
The name of the configuration to use. |
x |
|
Content |
Binary |
the document to encrypt |
|
|
Algorithm |
Enumeration, one of:
|
the algorithm for encryption |
|
|
Element Path |
String |
the path to the element to encrypt, if empty the whole document is considered |
|
|
Streaming Strategy |
|
Configure if repeatable streams should be used and their behavior |
|
|
Key Id |
String |
The key ID, as defined in the JCE configuration. |
|
|
Jce Key Info |
An inline key definition. |
|
||
Target Variable |
String |
The name of a variable on which the operation's output will be placed |
|
|
Target Value |
String |
An expression that will be evaluated against the operation's output and the outcome of that expression will be stored in the target variable |
|
|
Xml Sign Operation
<crypto:xml-sign>
Sign an XML document.
Parameters
Name | Type | Description | Default Value | Required |
---|---|---|---|---|
Configuration |
String |
The name of the configuration to use. |
x |
|
Content |
Binary |
the XML document to sign |
|
|
Digest Algorithm |
Enumeration, one of:
|
the hashing algorithm for signing |
|
|
Canonicalization Algorithm |
Enumeration, one of:
|
the canonicalization method for whitespace and namespace unification |
|
|
Type |
Enumeration, one of:
|
the type of signature to create |
|
|
Element Path |
String |
for internally detached signatures, an unambiguous XPath expression resolving to the element to sign |
|
|
Streaming Strategy |
|
Configure if repeatable streams should be used and their behavior |
|
|
Key Id |
String |
The key ID, as defined in the JCE configuration. |
|
|
Jce Key Info |
An inline key definition. |
|
||
Target Variable |
String |
The name of a variable on which the operation's output will be placed |
|
|
Target Value |
String |
An expression that will be evaluated against the operation's output and the outcome of that expression will be stored in the target variable |
|
|
Xml Validate Operation
<crypto:xml-validate>
Validate an XML signed document.
Parameters
Name | Type | Description | Default Value | Required |
---|---|---|---|---|
Configuration |
String |
The name of the configuration to use. |
x |
|
Content |
Binary |
Specifies the document to verify (includes the signature). |
|
|
Element Path |
String |
For internally detached signatures, an unambiguous XPath expression that resolves to the signed element. |
|
|
Use inline certificate if present |
Boolean |
Specify whether or not to validate the signature against a certificate contained in the |
|
|
Key Id |
String |
Specifies the key ID, as defined in the JCE configuration. |
|
|
Jce Key Info |
An inline key definition. |
|
Types Definition
Expiration Policy
Field | Type | Description | Default Value | Required |
---|---|---|---|---|
Max Idle Time |
Number |
A scalar time value for the maximum amount of time a dynamic configuration instance should be allowed to be idle before it’s considered eligible for expiration |
||
Time Unit |
Enumeration, one of:
|
A time unit that qualifies the maxIdleTime attribute |
Repeatable In Memory Stream
Field | Type | Description | Default Value | Required |
---|---|---|---|---|
Initial Buffer Size |
Number |
This is the amount of memory that will be allocated in order to consume the stream and provide random access to it. If the stream contains more data than can be fit into this buffer, then it will be expanded by according to the |
||
Buffer Size Increment |
Number |
This is by how much will be buffer size by expanded if it exceeds its initial size. Setting a value of zero or lower will mean that the buffer should not expand, meaning that a |
||
Max Buffer Size |
Number |
This is the maximum amount of memory that will be used. If more than that is used then a |
||
Buffer Unit |
Enumeration, one of:
|
The unit in which all these attributes are expressed |
Repeatable File Store Stream
Field | Type | Description | Default Value | Required |
---|---|---|---|---|
Max In Memory Size |
Number |
Defines the maximum memory that the stream should use to keep data in memory. If more than that is consumed then it will start to buffer the content on disk. |
||
Buffer Unit |
Enumeration, one of:
|
The unit in which maxInMemorySize is expressed |